はじめに
環境構築の記事を全面的に書き直しました。
違いは ESLint と Prettier を使用せず Biome を利用するようにしたことくらいです。
- 使用する技術スタック
- React
- TypeScript
- Vite
- pnpm
- Biome
目次
Node のインストール
asdf を利用して Node をインストールします。
1
2
3
4
|
$ asdf install nodejs 22.7.0
$ asdf global nodejs 22.7.0
$ node -v
v22.7.0
|
pnpm のインストール
npm ではなく pnpm を利用します。
1
2
3
|
$ npm i -g pnpm
$ pnpm -v
9.9.0
|
Vite で React x TypeScript を構築
1
2
3
4
5
|
$ cd $HOME
$ pnpm create vite myapp --template react-ts
$ cd myapp
$ pnpm install
$ pnpm run dev
|
Biome セットアップ
1
2
|
$ pnpm add --save-dev --save-exact @biomejs/biome
$ pnpm biome init # biome.json が生成される
|
biome.json
を編集します。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
{
"$schema": "https://biomejs.dev/schemas/1.8.3/schema.json",
"organizeImports": {
"enabled": true
},
"linter": {
"enabled": true,
"rules": {
"recommended": true
}
},
"formatter": {
"enabled": true,
"indentStyle": "space"
}
}
|
packages.json
を編集します。
1
2
3
|
- "lint": "eslint .",
+ "lint": "biome lint ./ && biome format ./",
+ "fix:lint": "biome lint --write ./ && biome format --write ./",
|
実行は以下のようにします。
1
2
|
$ pnpm run lint
$ pnpm run fix:lint # ファイルを書き換える
|
VSCode 設定
VSCode 拡張機能 Biome をインストールします。
settins.json
を編集します
1
2
3
4
5
6
7
8
9
10
11
12
13
|
...
"[json]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "biomejs.biome"
},
"[javascript][javascriptreact][typescript][typescriptreact]": {
"editor.formatOnSave": true,
"editor.defaultFormatter": "biomejs.biome",
"editor.codeActionsOnSave": {
"quickfix.biome": "explicit"
}
},
...
|
ESLint 削除
2024年9月現在 Vite でセットアップを行うと初期から eslint プラグインと設定ファイルが存在します。
Biome を使うため削除します。
1
2
|
$ rm eslint.config.js
$ pnpm uninstall @eslint/js eslint eslint-plugin-react-hooks eslint-plugin-react-refresh typescript-eslint
|
絶対パスでインポート
import などを行うときに相対パスではなく絶対パスで行いたいので vite.config.ts
に以下を設定します。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
+import path from 'path'
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react()],
+ root: './',
+ resolve: {
+ alias: {
+ '@/': path.join(__dirname, './src/'),
+ },
+ },
})
|
tsconfig.json
に追記します。
多くの設定で baseUrl
が書かれていますが、昔のバージョン(4.0以前)の名残なので今は必要ありません。
1
2
3
4
5
|
+ "compilerOptions": {
+ "paths": {
+ "@/*": ["./src/*"]
+ }
+ }
|
こちらも2024年9月現在 Vite でセットアップを行うと ./tsconfig.app.json
と ./tsconfig.node.json
を読み込むような設定になっています。
compilerOptions
で内容が重複してうまく動作しないので両方のファイルの先頭に extends
を追加します。
1
2
3
4
5
6
|
{
"extends": "./tsconfig.json",
"compilerOptions": {
...
}
}
|
また下記のようなエラーが出る場合は $ npm i -D @types/node
とすることで解消されます。
Cannot find module 'path' or its corresponding type declarations.
Cannot find name '__dirname'.
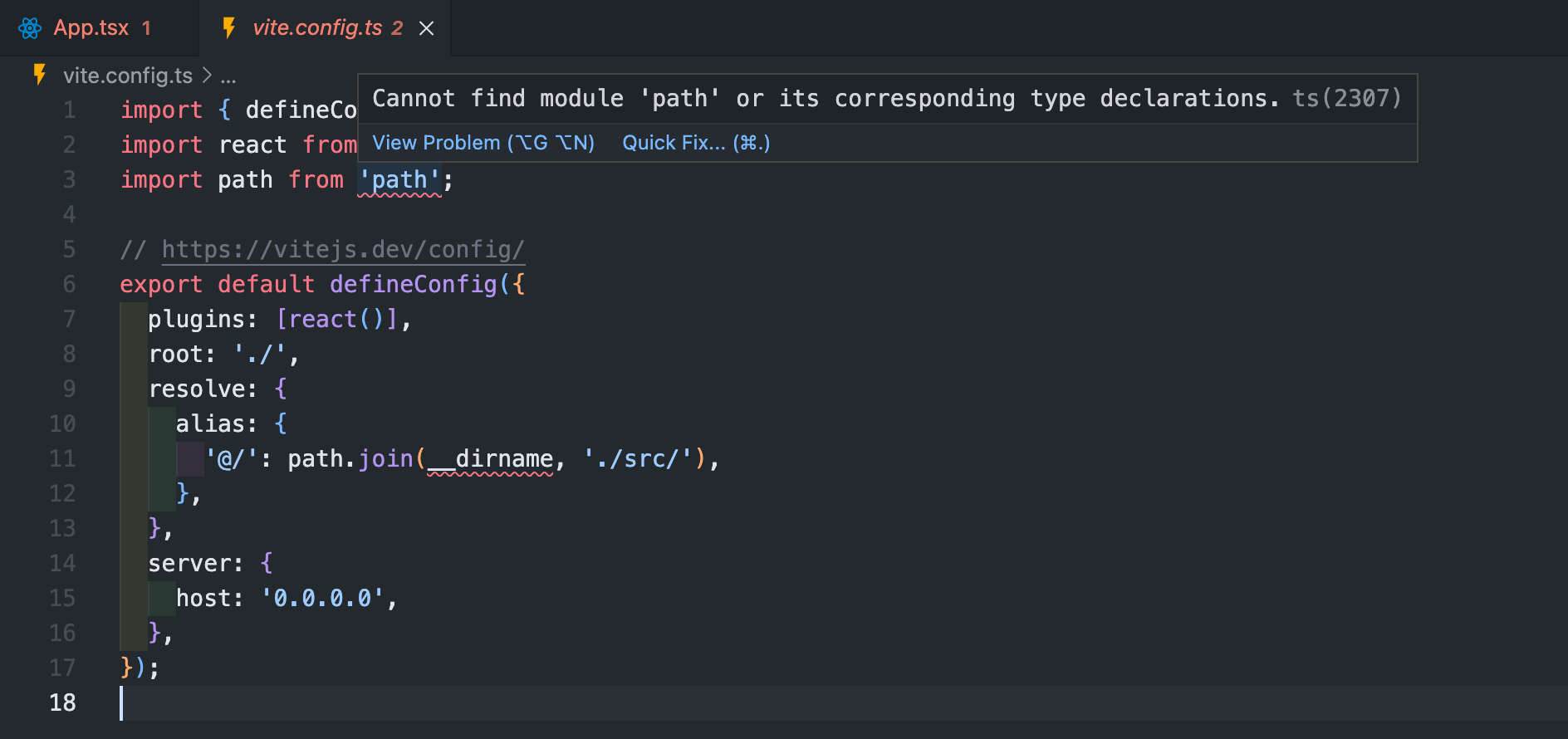
Tips として vite.config.ts
と tsconfig.json
で同じようなパスの設定を書いてますが、次のような理由があるためです。
Vite では tsconfig.json
にパスを書いても無視されてしまいます。
Vite に認識してもらうために vite.config.ts
にパスの設定を書きます。
tsconfig.json
でパスの設定をしている理由は VSCode などを使っているときにパスが読み込めるようにするためというエディター側に認識させる都合上書いています。
そのため Vite ではなく create-react-app
で作成したアプリの場合は tsconfig.json
に path の設定を書くだけで大丈夫です。